QGraphicsItemAnimation::timeLine() vs QPropertyAnimation:比較と使い分け
Qt WidgetsにおけるQGraphicsItemAnimation::timeLine()の詳細解説
QGraphicsItemAnimation::timeLine()
は、Qt Widgetsフレームワークにおけるアニメーション機能の一つで、時間経過に伴ってQGraphicsItemの状態を変化させるための強力なツールです。この関数を使用することで、直感的なコードで複雑なアニメーションを作成することができます。
機能
QGraphicsItemAnimation::timeLine()
は以下の機能を提供します:
- 時間経過に伴う属性の変化:
- 位置、サイズ、回転、不透明度など、様々な属性をアニメーション化できます。
- easing曲線による滑らかな動き:
- 動きを滑らかにしたり、加速/減速させたりするeasing曲線を適用できます。
- ループ再生:
- アニメーションを一定回数または無限にループさせることができます。
- シグナル/スロットによる制御:
- アニメーションの状態変化をシグナル/スロットを使って監視・制御できます。
コード例
以下のコード例は、QGraphicsItemAnimation::timeLine()
を使って、円形に移動するQGraphicsItemを作成する方法を示します:
#include <Qt>
class Example : public QGraphicsView {
QGraphicsScene scene;
QGraphicsItem *item;
QGraphicsItemAnimation *animation;
public:
Example() {
setScene(&scene);
item = new QGraphicsRectItem(0, 0, 100, 100);
scene.addItem(item);
// タイムラインを作成
animation = new QGraphicsItemAnimation(item);
// アニメーションの開始と終了時間を設定
animation->setTimeLine(QTimeLine(1000));
// キーフレームを設定
animation->setKeyFrame(0, QPointF(0, 0));
animation->setKeyFrame(0.5, QPointF(50, 50));
animation->setKeyFrame(1, QPointF(0, 0));
// イージング曲線を設定
animation->setEasingCurve(QEasingCurve::InOutQuad);
// アニメーションを開始
animation->start();
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
Example example;
example.show();
return app.exec();
}
詳細解説
QGraphicsItemAnimation
オブジェクトを作成し、アニメーションさせたいQGraphicsItemを渡します。QTimeLine
オブジェクトを作成し、アニメーションの長さを設定します。setKeyFrame()
を使って、アニメーションの開始点、中間点、終点におけるQGraphicsItemの状態を設定します。setEasingCurve()
を使って、アニメーションの動きを制御するeasing曲線を設定します。start()
を使ってアニメーションを開始します。
補足
- 上記のコード例は基本的な使い方を示しています。より複雑なアニメーションを作成するには、
QGraphicsItemAnimation
クラスの他の機能も活用できます。 - Qtには、
QPropertyAnimation
など、QGraphicsItemAnimation
以外にもアニメーションを作成するためのクラスが用意されています。
QGraphicsItemAnimation::timeLine()
について、さらに詳しく知りたい場合は、お気軽に質問してください。
Qt WidgetsにおけるQGraphicsItemAnimation::timeLine()のサンプルコード集
#include <Qt>
class Example : public QGraphicsView {
QGraphicsScene scene;
QGraphicsItem *item;
QGraphicsItemAnimation *animation;
public:
Example() {
setScene(&scene);
item = new QGraphicsRectItem(0, 0, 100, 100);
scene.addItem(item);
// タイムラインを作成
animation = new QGraphicsItemAnimation(item);
// アニメーションの開始と終了時間を設定
animation->setTimeLine(QTimeLine(1000));
// キーフレームを設定
animation->setKeyFrame(0, QPointF(0, 0));
animation->setKeyFrame(0.5, QPointF(50, 50));
animation->setKeyFrame(1, QPointF(0, 0));
// イージング曲線を設定
animation->setEasingCurve(QEasingCurve::InOutQuad);
// アニメーションを開始
animation->start();
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
Example example;
example.show();
return app.exec();
}
拡縮アニメーション
#include <Qt>
class Example : public QGraphicsView {
QGraphicsScene scene;
QGraphicsItem *item;
QGraphicsItemAnimation *animation;
public:
Example() {
setScene(&scene);
item = new QGraphicsRectItem(0, 0, 100, 100);
scene.addItem(item);
// タイムラインを作成
animation = new QGraphicsItemAnimation(item);
// アニメーションの開始と終了時間を設定
animation->setTimeLine(QTimeLine(1000));
// キーフレームを設定
animation->setKeyFrame(0, QRectF(0, 0, 100, 100));
animation->setKeyFrame(0.5, QRectF(25, 25, 50, 50));
animation->setKeyFrame(1, QRectF(0, 0, 100, 100));
// イージング曲線を設定
animation->setEasingCurve(QEasingCurve::InOutQuad);
// アニメーションを開始
animation->start();
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
Example example;
example.show();
return app.exec();
}
回転アニメーション
#include <Qt>
class Example : public QGraphicsView {
QGraphicsScene scene;
QGraphicsItem *item;
QGraphicsItemAnimation *animation;
public:
Example() {
setScene(&scene);
item = new QGraphicsRectItem(0, 0, 100, 100);
scene.addItem(item);
// タイムラインを作成
animation = new QGraphicsItemAnimation(item);
// アニメーションの開始と終了時間を設定
animation->setTimeLine(QTimeLine(1000));
// キーフレームを設定
animation->setKeyFrame(0, 0.0);
animation->setKeyFrame(0.5, 90.0);
animation->setKeyFrame(1, 0.0);
// イージング曲線を設定
animation->setEasingCurve(QEasingCurve::InOutQuad);
// アニメーションを開始
animation->start();
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
Example example;
example.show();
return app.exec();
}
複数のアニメーションを組み合わせる
#include <Qt>
class Example : public QGraphicsView {
QGraphicsScene scene;
QGraphicsItem *item;
QGraphicsItemAnimation *animation1;
QGraphicsItemAnimation *animation2;
public:
Example() {
setScene(&scene);
item = new QGraphicsRectItem(0, 0, 100, 100);
scene.addItem(item);
// タイムラインを作成
animation1
Qt WidgetsにおけるQGraphicsItemのアニメーション作成方法
QPropertyAnimation
は、QGraphicsItemのプロパティを時間経過に伴って変化させるアニメーションを作成するためのクラスです。QGraphicsItemAnimation::timeLine()
よりもシンプルで軽量なアニメーションを作成したい場合に適しています。
コード例
#include <Qt>
class Example : public QGraphicsView {
QGraphicsScene scene;
QGraphicsItem *item;
QPropertyAnimation *animation;
public:
Example() {
setScene(&scene);
item = new QGraphicsRectItem(0, 0, 100, 100);
scene.addItem(item);
// アニメーションを作成
animation = new QPropertyAnimation(item, "pos");
// アニメーションの開始と終了時間を設定
animation->setDuration(1000);
// キーフレームを設定
animation->setStartValue(QPointF(0, 0));
animation->setEndValue(QPointF(50, 50));
// イージング曲線を設定
animation->setEasingCurve(QEasingCurve::InOutQuad);
// アニメーションを開始
animation->start();
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
Example example;
example.show();
return app.exec();
}
QSequentialAnimationGroup
は、複数のアニメーションを順番に実行するためのクラスです。複雑なアニメーションを作成したい場合に適しています。
コード例
#include <Qt>
class Example : public QGraphicsView {
QGraphicsScene scene;
QGraphicsItem *item;
QSequentialAnimationGroup *group;
QPropertyAnimation *animation1;
QPropertyAnimation *animation2;
public:
Example() {
setScene(&scene);
item = new QGraphicsRect
Qt GUIアプリケーションでカーソルに関するイベントを処理する
この関数の使いどころ特定のウィンドウ上でマウス操作を無効化したい場合独自のカーソル画像を表示したい場合画面全体に表示されるウィンドウを作成する場合コード例この関数の注意点QWindow::unsetCursor()は、ウィンドウ全体に適用されます。特定のウィジェット内でのみカーソルを非表示にする場合は、QWidget::setCursor(Qt::BlankCursor)などの他の方法を使用する必要があります。
QPainter::restore() をマスターして、Qt GUI プログラミングをレベルアップ
QPainter::restore() は、直前にQPainter::save() で保存した描画状態を復元します。具体的には、以下の設定が復元されます。ペン:色、幅、スタイル、描画モードなどブラシ:色、スタイル、描画モードなど座標変換:ワールド座標系とウィジェット座標系の変換
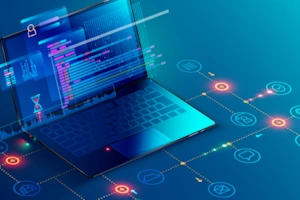
Qt GUI での折れ線描画:QPainter::drawPolyline() 関数の使い方
使い方この関数は、以下の引数を受け取ります。painter: 描画対象となる QPainter オブジェクトpoints: 折れ線の頂点を表す QPoint または QPointF 型の配列pointCount: 配列 points の要素数
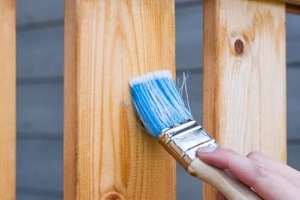
QTextTableFormat::setAlignment() 関数の使い方
QTextTableFormat::setAlignment() は、Qt GUI でテキストテーブルの配置を設定するために使用する関数です。この関数は、テーブル内のテキストを水平方向と垂直方向にどのように配置するかを指定します。引数alignment : テキストの配置を指定する Qt::Alignment 型の値。
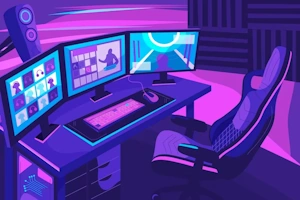
Qt GUI アプリケーション開発でアイコンサイズを自在に操る!QIcon::availableSizes() 関数徹底解説
概要:QIcon クラスは、Qt GUI アプリケーションで使用されるアイコンを表します。availableSizes() 関数は、QIcon オブジェクトに対して呼び出すことができます。この関数は、QSize 型のオブジェクトのリストを返します。
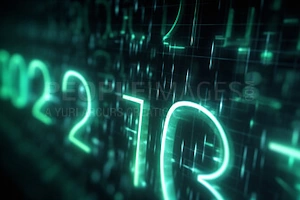

Qt WidgetsフレームワークにおけるQGraphicsView::event()の役割
**QGraphicsView::event()**関数は、イベント処理の起点となる重要な役割を担います。この関数は、以下のステップでイベントを処理します。イベントの受け取り:QGraphicsView::event()関数は、ウィジェットに送信されたイベントを受け取ります。
Qt Widgetsで柔軟なデータマッピング:QDataWidgetMapper::setItemDelegate()の使い方
QDataWidgetMapper::setItemDelegate()は、モデル内のデータとウィジェット間のマッピングに柔軟性と高度なカスタマイズ性を追加する強力な関数です。この関数は、個々のウィジェットに独自のデリゲートを設定することで、データ表示と編集の処理をより細かく制御できます。
Qt WidgetsにおけるQProxyStyle::layoutSpacing()とは
QProxyStyle::layoutSpacing()は、Qt Widgetsで使用される関数で、ウィジェット間のレイアウトスペースを取得するために使用されます。この関数は、ウィジェット間のデフォルトの水平方向および垂直方向のスペースをピクセル単位で返します。
QGraphicsView::render() 関数のサンプルコード
QGraphicsView::render() は、Qt Widgetsフレームワークの一部である QGraphicsView クラスの重要な関数です。この関数は、ビュー内のグラフィックスシーンを画像としてレンダリングし、さまざまな形式で保存したり、他のアプリケーションと共有したりすることができます。
QOpenGLExtraFunctions::glProgramUniform4uiv() 関数解説
QOpenGLExtraFunctions::glProgramUniform4uiv() 関数は、Qt GUIアプリケーションでOpenGLプログラムのユニフォーム変数に4つの無符号整数を設定するために使用されます。この関数は、Qt 5.15以降で導入されました。